AccordionControl是一种高级导航控件,允许您创建紧凑且易于导航的分层用户界面。
其功能包括:
- 无限数量的层次结构
- 多种选择模式
- 数据绑定支持
- 多种扩展模式
- 展开/折叠动画
- 支持Glyph
- 自定义项目内容
- 综合搜索领域
- 键盘导航
提供有关如何使用AccordionControl创建简单应用程序的逐步说明如下。
步骤1.添加数据模型
1.AccordionControl可以绑定到实现IEnumerable接口的任何对象或其后代(例如,IList、ICollection)
下面的代码示例演示了本教程中使用的简单数据模型:
using System.Collections.Generic; namespace DxAccordionGettingStarted { public class Employee { public int ID { get; set; } public string Name { get; set; } public string Position { get; set; } public string Department { get; set; } public override string ToString() { return Name; } } public static class Stuff { public static List<Employee> GetStuff() { List<Employee> stuff = new List<Employee>(); stuff.Add(new Employee() { ID = 1, Name = "Gregory S. Price", Department = "Management", Position = "President" }); stuff.Add(new Employee() { ID = 2, Name = "Irma R. Marshall", Department = "Marketing", Position = "Vice President" }); stuff.Add(new Employee() { ID = 3, Name = "John C. Powell", Department = "Operations", Position = "Vice President" }); stuff.Add(new Employee() { ID = 4, Name = "Christian P. Laclair", Department = "Production", Position = "Vice President" }); stuff.Add(new Employee() { ID = 5, Name = "Karen J. Kelly", Department = "Finance", Position = "Vice President" }); stuff.Add(new Employee() { ID = 6, Name = "Brian C. Cowling", Department = "Marketing", Position = "Manager" }); stuff.Add(new Employee() { ID = 7, Name = "Thomas C. Dawson", Department = "Marketing", Position = "Manager" }); stuff.Add(new Employee() { ID = 8, Name = "Angel M. Wilson", Department = "Marketing", Position = "Manager" }); stuff.Add(new Employee() { ID = 9, Name = "Bryan R. Henderson", Department = "Marketing", Position = "Manager" }); stuff.Add(new Employee() { ID = 10, Name = "Harold S. Brandes", Department = "Operations", Position = "Manager" }); stuff.Add(new Employee() { ID = 11, Name = "Michael S. Blevins", Department = "Operations", Position = "Manager" }); stuff.Add(new Employee() { ID = 12, Name = "Jan K. Sisk", Department = "Operations", Position = "Manager" }); stuff.Add(new Employee() { ID = 13, Name = "Sidney L. Holder", Department = "Operations", Position = "Manager" }); stuff.Add(new Employee() { ID = 14, Name = "James L. Kelsey", Department = "Production", Position = "Manager" }); stuff.Add(new Employee() { ID = 15, Name = "Howard M. Carpenter", Department = "Production", Position = "Manager" }); stuff.Add(new Employee() { ID = 16, Name = "Jennifer T. Tapia", Department = "Production", Position = "Manager" }); stuff.Add(new Employee() { ID = 17, Name = "Judith P. Underhill", Department = "Finance", Position = "Manager" }); stuff.Add(new Employee() { ID = 18, Name = "Russell E. Belton", Department = "Finance", Position = "Manager" }); return stuff; } } }
步骤2-3.添加视图模型
2.创建从数据模型中检索数据的视图模型:
using System.Collections.Generic; using System.Collections.ObjectModel; using System.Linq; namespace DxAccordionGettingStarted { public class MainWindowViewModel { public MainWindowViewModel() { var employeeDepartments = Stuff.GetStuff() .GroupBy(x => x.Department) .Select(x => new EmployeeDepartment(x.Key, x.ToArray())); EmployeeDepartments = new ObservableCollection<EmployeeDepartment>(employeeDepartments.ToArray()); } public ObservableCollection<EmployeeDepartment> EmployeeDepartments { get; set; } } public class EmployeeDepartment { public string Name { get; set; } public ObservableCollection<Employee> Employees { get; set; } public EmployeeDepartment(string name, IEnumerable<Employee> employees) { Name = name; Employees = new ObservableCollection<Employee>(employees); } public override string ToString() { return Name; } } }
3.构建解决方案。调用主窗口的智能标记并定义其数据上下文,如下图所示:
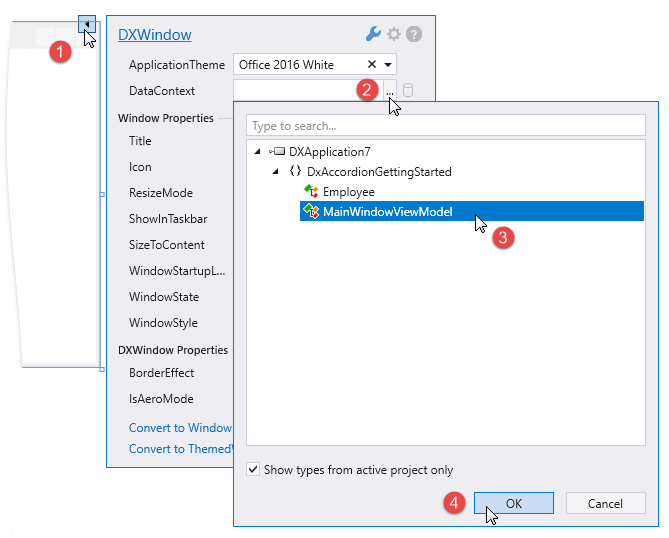
步骤4-5.将AccordionControl添加到视图
4.从DX.18.2:导航和布局工具箱选项卡中拖动AccordionControl并将其放到主窗口:
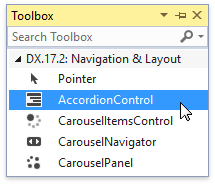
5.右键单击该控件并选择Layout|Reset All以允许AccordionControl填充整个窗口:
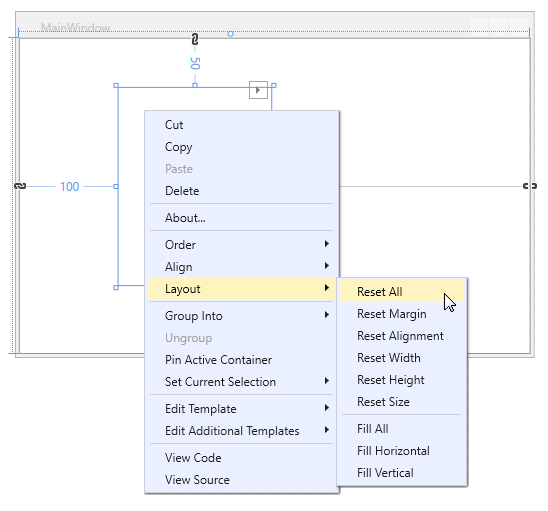
步骤6-7.两个数据集的AccordionControl
6.调用accordioncontrol的tag去定义ItemsSource field:
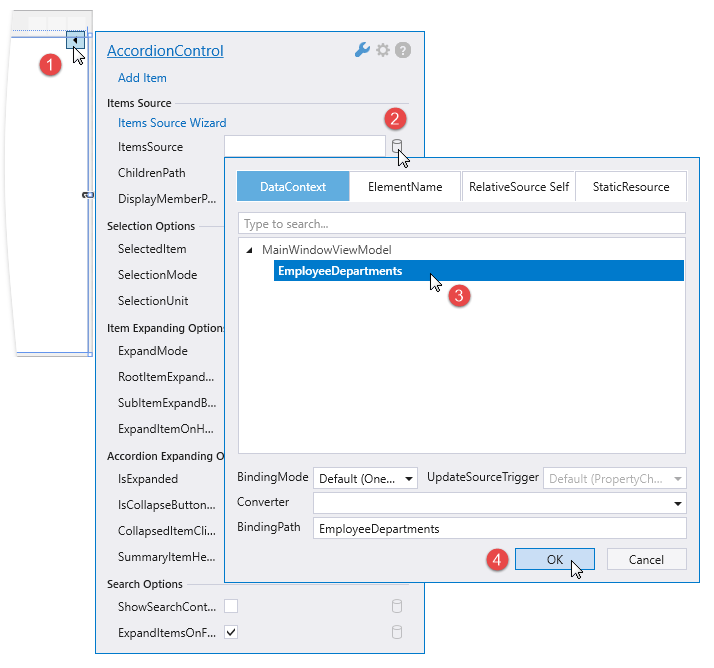
7.定义ChildrenPath字段,该字段指定包含子项的属性的路径:
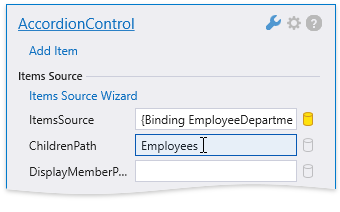
下面的代码示例演示生成的代码:
<dx:DXWindow xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:dx="http://schemas.devexpress.com/winfx/2008/xaml/core" xmlns:dxb="http://schemas.devexpress.com/winfx/2008/xaml/bars" xmlns:dxa="http://schemas.devexpress.com/winfx/2008/xaml/accordion" xmlns:local="clr-namespace:DxAccordionGettingStarted" x:Class="DxAccordionGettingStarted.MainWindow" Title="MainWindow" Height="350" Width="525"> <dx:DXWindow.DataContext> <local:MainWindowViewModel/> </dx:DXWindow.DataContext> <Grid> <dxa:AccordionControl ItemsSource="{Binding EmployeeDepartments}" ChildrenPath="Employees"/> </Grid> </dx:DXWindow>
步骤8.得到结果
8.运行解决方案以查看结果:
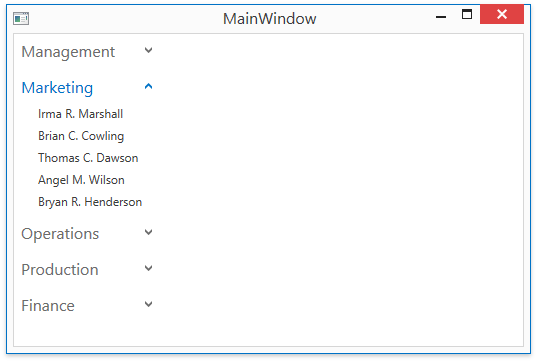
买 DevExpress Universal Subscription 免费赠 万元汉化资源包1套!
限量15套!先到先得,送完即止!立即抢购>>
欢迎任何形式的转载,但请务必注明出处,尊重他人劳动成果
转载请注明:文章转载自:DevExpress控件中文网 [https://www.devexpresscn.com/]
本文地址:https://www.devexpresscn.com/post/1200.html